Seven Zip Java Example
Compressing and Decompressing Data Using Java APIs by Qusay H. Mahmoud Published February 2002 Many sources of information contain redundant data or data that adds little to the stored information. This results in tremendous amounts of data being transferred between client and server applications or computers in general. The obvious solution to the problems of data storage and information transfer is to install additional storage devices and expand existing communication facilities. To do so, however, requires an increase in an organization's operating costs.
One method to alleviate a portion of data storage and information transfer is through the representation of data by more efficient code. This article presents a brief introduction to data compression and decompression, and shows how to compress and decompress data, efficiently and conveniently, from within your Java applications using the java.util.zip package. While it is possible to compress and decompress data using tools such as WinZip, gzip, and Java ARchive (or jar), these tools are used as standalone applications. It is possible to invoke these tools from your Java applications, but this is not a straightforward approach and not an efficient solution.
22 example code snippets; Over 6700. The native part of 7-Zip-JBinding communicates with the java part through the java jni interface and uses the corresponding.
- Download free Seven Zip Java Example. I read the example Client7z files and I managed to add basic compression and. Doc)sevenzip- jbinding.
- I want to extract some files from a 7-zip byte stream,it can't be stored on hard disk,so I can't. How to extract files from a 7-zip stream in Java without store.
Give More Feedback
This is especially true if you wish to compress and decompress data on the fly (before transferring it to a remote machine for example). This article:. Gives you a brief overview of data compression. Describes the java.util.zip package. Shows how to use this package to compress and decompress data. Shows how to compress and decompress serialized objects to save disk space. Shows how to compress and decompress data on the fly to improve the performance of client/server applications Overview of Data Compression The simplest type of redundancy in a file is the repetition of characters.
For example, consider the following string: BBBBHHDDXXXXKKKKWWZZZZ This string can be encoded more compactly by replacing each repeated string of characters by a single instance of the repeated character and a number that represents the number of times it is repeated. The earlier string can be encoded as follows: 4B2H2D4X4K2W4Z Here '4B' means four B's, and 2H means two H's, and so on. Compressing a string in this way is called run-length encoding. As another example, consider the storage of a rectangular image. As a single color bitmapped image, it can be stored as shown in Figure 1.
Figure 1: A bitmap with information for run-length encoding Another approach might be to store the image as a graphics metafile: Rectangle 11, 3, 20, 5 This says, the rectangle starts at coordinate (11, 3) of width 20 and length 5 pixels. The rectangular image can be compressed with run-length encoding by counting identical bits as follows: 0, 40 0, 40 0,10 1,20 0,10 0,10 1,1 0,18 1,1 0,10 0,10 1,1 0,18 1,1 0,10 0,10 1,1 0,18 1,1 0,10 0,10 1,20 0,10 0,40 The first line above says that the first line of the bitmap consists of 40 0's.
The third line says that the third line of the bitmap consists of 10 0's followed by 20 1's followed by 10 more 0's, and so on for the other lines. Note that run-length encoding requires separate representations for the file and its encoded version.
Therefore, this method cannot work for all files. Other compression techniques include variable-length encoding (also known as Huffman Coding), and many others. For more information, there are many books available on data and image compression techniques. There are many benefits to data compression.
The main advantage of it, however, is to reduce storage requirements. Also, for data communications, the transfer of compressed data over a medium results in an increase in the rate of information transfer.
Note that data compression can be implemented on existing hardware by software or through the use of special hardware devices that incorporate compression techniques. Figure 2 shows a basic data-compression block diagram.
Figure 2: Data-compression block diagram ZIP vs. GZIP If you are working on Windows, you might be familiar with the WinZip tool, which is used to create a compressed archive and to extract files from a compressed archive. On UNIX, however, things are done a bit differently. The tar command is used to create an archive (not compressed) and another program ( gzip or compress) is used to compress the archive. Tools such as WinZip and PKZIP act as both an archiver and a compressor. They compress files and store them in an archive. On the other hand, gzip does not archive files.
Therefore, on UNIX, the tar command is usually used to create an archive then the gzip command is used to compress the archived file. The java.util.zip Package Java provides the java.util.zip package for zip-compatible data compression. It provides classes that allow you to read, create, and modify ZIP and GZIP file formats. It also provides utility classes for computing checksums of arbitrary input streams that can be used to validate input data. This package provides one interface, fourteen classes, and two exception classes as shown in Table 1. Table 1: The java.util.zip package Item Type Description Checksum Interface Represents a data checksum. Note: Another fundamental difference between ZIPInputStream and ZipFile is in terms of caching.
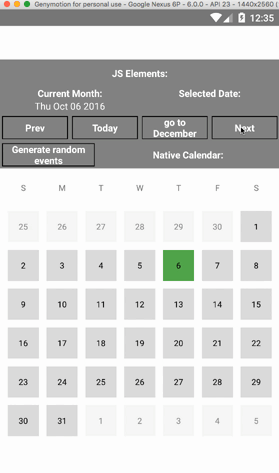
Zip entries are not cached when the file is read using a combination of ZipInputStream and FileInputStream. However, if the file is opened using ZipFile(fileName) then it is cached internally, so if ZipFile(fileName) is called again the file is opened only once. The cached value is used on the second open. If you work on UNIX, it is worth noting that all zip files opened using ZipFile are memory mapped, and therefore the performance of ZipFile is superior to ZipInputStream. If the contents of the same zip file, however, are be to frequently changed and reloaded during program execution, then using ZipInputStream is preferred.